What are Data Structure & Variables?
Hello programmers, so today we are going to learn about data structure and variables in python. Data structures are a way of organizing our data on the computer it's very much like organizing our things in our living hall or room Consider an example that you have a collection of books of different authors and genres and you want to keep all your books organized on the bookshelf so that it looks neatπ and easier for you to get whatever book you want. Suppose your bookshelf looks something like this.
Now for the ease of finding your books you numbered the position of each book placed on your bookshelf. Something like this:
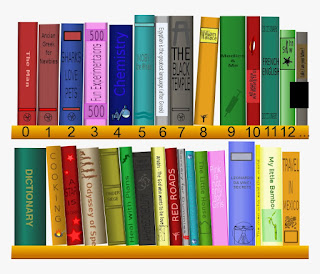
Here you have created a data structure for yourself in your home. Smart move!!π and since you are pretty intelligent you can read the label of your book or you just know that your chemistry book is dark blue in color. But that not the case for me(@python). As you see, I don't know how to read to get English and that to from the computer. And Believe me, that thing only understands the language of binary or 0s and 1s. So it is difficult to communicate with computers in English but we could come up with a strategy so that both you and I can communicate with it. Suppose you asked me to get the book in the 4th position on your bookshelf I would do it without any hesitation π. As you know it is easier for me to interpret numbers to binary. There are various ways in which you could store your data on the computer and it is easier for me to access it for you. Example, you could store it in a Key:Value pair where the value is your data and the key is a unique number to access it. So, to sum up, a data structure is nothing but a structure to store your data on the computer, and in this case, your bookshelf would be your data structure and your books are your data. There are different kinds of data structure that python uses like:1. Lists
2. Dictionary
3. Sets
and I will be explaining all of these to you. But before that, "do you know what is data"?
Data is nothing but information stored in a system/computer. Like your book which contains specific facts and knowledge about a story or a lesson. Although the computer stores data in a different manner it stores it in a binary format(0s_and_1s) and believe me it's difficult to understand that. But, you don't need to worry about that as I will handle itπ. You just need to instruct me that way I am going to ask you to. So, we are going to start our lesson here.
What is a Variable?
Variable is a reserved memory location on the computer. Now, a reserved memory location on a computer is like your space in your bookshelf for each book. Something like this:
Here each small box in your bookshelf can be called a memory location I have marked M1, M2, M2......M18 so, that it is easier for you to understand. And each small box of your bookshelf contains a book that has certain information which is our data. Also, you can add/remove or replace data from your memory locations in your computer same as adding/removing or replacing books from your bookshelf. But to use a variable you have to declare it first on your computer.
What is the Variable Declaration?
Variable declaration is nothing but informing the computer that we are going to need a memory location to store our data and we would name the memory location using different variable names.
In the python code as shown above. Here x and y is your variable name having values 9 and 22.
Now, the computer stores data in different formats or types such as integer, float, string, etc. these are known as data types in python.
What are Data types?
Data types are basically the different types or classifications of data items.
Such as an integer(0,1,2..n) or string(sequence of characters). To understand these consider an example in your daily life you classify your information so that it is easy for you to memorize it. Such as, you know that a phone number will always be in integer you will not mistake a phone number to be alphabets or string as we say in python. In the same way, even python classifies its data so that it is easier for us to access or modify our data There are different types of data types in Python and these can be classified as:
"We are going to go through each data types in python"
- Numeric: It represents all data having numeric value. A numeric value can be classified as:
1.Integer: It contains positive or negative whole numbers without fraction or decimal(Eg. 1,2,5 etc.).
2.Float: It contains real numbers with fraction or decimal and has floating point representation Eg(1.2,1.345,3.14 etc.).
3.Complex: It contains two parts which are classified as real part and imaginary part(Eg. x+yj). These are usually used for mathematical calculation.
- Sequence: Sequence is the ordered collection of similar or different data types. Sequences allow storing multiple values in an organized manner. There are several sequence types in Python β
1. String: Strings are arrays of bytes representing Unicode characters. A string is a collection of one or more characters put in a single quote(' '), double-quote(" ") or triple quote.
For example, "Apple", "BMW" etc. anything that with a sequence of characters. In this case, the characters are English alphabet.And each character are indexed for better access as shown:
2. Lists: Lists are just like the arrays, declared in other languages which is a ordered collection of data. It is very flexible as the items in a list do not need to be of the same data type.
In python list is written as:
List = ["Pen", "Pencil", "Notebook"]
3. Tuple: A tuple is a collection of objects which ordered and immutable. Tuples are sequences, just like lists. The differences between tuples and lists are, the tuples cannot be changed unlike lists.
In python tuple is written as:
Tuple = ("Pen", "Pencil", "Notebook")
- Boolean: The boolean data type is either True or False. In Python, boolean variables are defined by the True and False keywords
- Sets: A set is an unordered collection of items. Every set element is unique (no duplicates) and must be immutable (cannot be changed). However, a set itself is mutable. We can add or remove items from it. Sets can also be used to perform mathematical set operations like union, intersection, symmetric difference, etc.
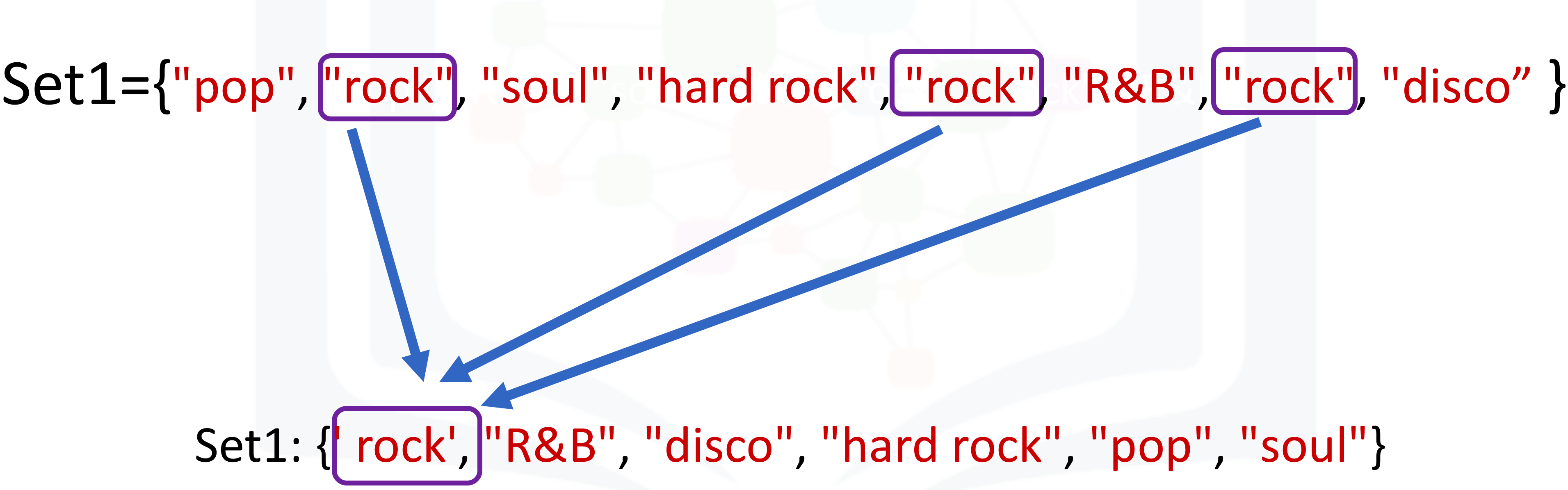
- Dictionary: A dictionary is a collection that is unordered, changeable, and indexed. In Python, dictionaries are written with curly brackets, and they have keys and value.
Abstract Data types:
Before defining abstract data types, let us consider the different views of system-defined data types. We all know that, by default, all primitive data types (int, float, etc.) support basic operations such as addition and subtraction. The system provides the implementations for the primitive data types. For user-defined data types, we also need to define operations. The implementation for these operations can be done when we want to actually use them. That means, in general, user-defined data types are defined along with their operations. To simplify the process of solving problems, we combine the data structures with their operations
and we call this Abstract Data Types (ADTs). An ADT consists of two parts:
1. Declaration of data
2. Declaration of operations
Commonly used ADTs include Linked Lists, Stacks, Queues, Priority Queues, Binary Trees, Dictionaries, Disjoint Sets (Union and Find), Hash Tables, Graphs, and many others. For example, the stack uses the LIFO (Last-In-First-Out) mechanism while storing the data in data structures. The last element inserted into the stack is the first element that gets deleted. Common operations of it are: creating the stack, pushing an element onto the stack, popping an element from the stack, finding the current top of the stack, finding the number of elements in the stack, etc. While defining the ADTs do not worry about the implementation details. They come into the picture only when we want to use them. Different kinds of ADTs are suited to different kinds of applications, and some are highly specialized to specific tasks.
And that's all you needed to know about data structures and data types in python. Now we are going to use these data structures and do some awesome stuff in python π
No comments:
Post a Comment